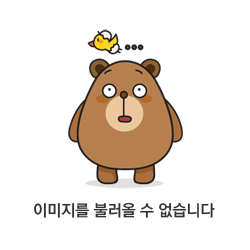
프로토 타입 도식
new 연산자를 호출하면 Constructor에 정의 한대로 인스턴스가 생성됨
그리고 instance에 __proto__라는 프로퍼티가 자동으로 부여되는데 __proto__ 프로퍼티 Constructor의 prototpye 프로터티를 참조함
var Person = function (name) {
this._name = name;
}
Person.prototype.getName = function() {
return this._name;
}
var suzi = new Person("Suzi");
// this 가 제대로 바인딩 되지 않음.
// this가 suzi.__proto__를 가르키고 있음.
console.log(suzi.__proto__.getName()); // undefined
console.log(Person.prototype === suzi.__proto__) // true;
//__proto__는 생략이 가능한 인스턴스임.
// 생략하지 않으면 suzi.__proto__ 를 가르키지만
// 생략하면 suzi 를 가르킨다.
console.log(suzi.getName()); // Suzi;
함수의 prototype에 어떤 메서드나 프로퍼티가 있따면 인스턴스에서도 마치 자신의 것처럼 해당 메서드, 프로퍼티를 사용할수 있다.
var arr = [1,2];
console.dir(arr);
console.dir(Array);
arr.forEach(function (){});
Array.isArray(arr); // true
arr.isArray(); // TypeError 프로토타입에 내부에 있지 않음
constructor 프로퍼티
prototype 객체 내부 에 있음. 원래의 생성자 함수를 참조
인스턴스로부터 그 원형이 무엇인지 알수 있는 수단이 된다.
var arr = [1,.2];
Array.prototype.constructor === Array; // true
arr.__proto__.constructor === Array; // true
arr.constructor === Array; //true
var arr2 = new arr.constructor(3,4);
console.log(arr2); // [3,4];
인스턴스의 __proto__ 가 생성자 함수의 prototype 프로퍼티를 참조하며 __proto__가 생략 가능하므로 직접 constructor를 접근할수 있다.
프로토타입 체이닝
const Person = function (name){
this.name = name;
}
Person.prototype.getName = function() {
return this.name;
}
const iu = new Person('부정수');
iu.getName = function () {
return this.name + "변경";
}
//인스턴스가 동일한 프로퍼티, 메서드를 가지고 있는 상황
// prototype.getName이 오버라이드된다.
// 자신의 프로퍼티에서 찾고 없으면 그다음으로 가까운 대상에서 찾는다.
console.log(iu.getName()) // 부정수 변경;
어떤 데이터의 __proto__ 프로퍼티 내부에 다시 __proto__ 프로퍼티가 연쇄적으로 이어진 적을 프로토타입 체인이라고 부르고, 이 체인을 따라가며 검색하는 것을 프로토타입 체이닝이라고 한다.
어떤 생성자 함수이든 prototype 은 반드시 객체이므로 최상단에 Object.prototype 이 존재한다.
그러므로 전역적으로 (어떤 데이터를 대상으로도) 사용할수 있을법만 메서드만 __proto__에 정의해야한다.
ex) toSTring, hasOwnPrperty, valueOf,
그 외는 Object.static 메서드로 정의해야한다.
다중 프로토타입 체인