1. ThreadLocal 이란?
Java.lang 패키지에서 제공하는 쓰레드 스코프 데이터 저장소
같은 쓰레드 scope 내에서 데이터 공유
사용방법은 간단하다.
ThreadLocal를 객체를 선언하고
get(),set(),remove() 메소드를 이용하여 저장하면 된다.
package com.ssafy;
public class Test {
static ThreadLocal<String> threadLocal;
public static void main(String[] args) throws Exception {
threadLocal = new ThreadLocal<String>();
Thread thread1 = new Thread(()-> {
threadLocal.set("111");
System.out.println(threadLocal.get());
});
Thread thread2 = new Thread(()-> {
threadLocal.set("222");
System.out.println(threadLocal.get());
});
thread1.start();
thread2.start();
}
}
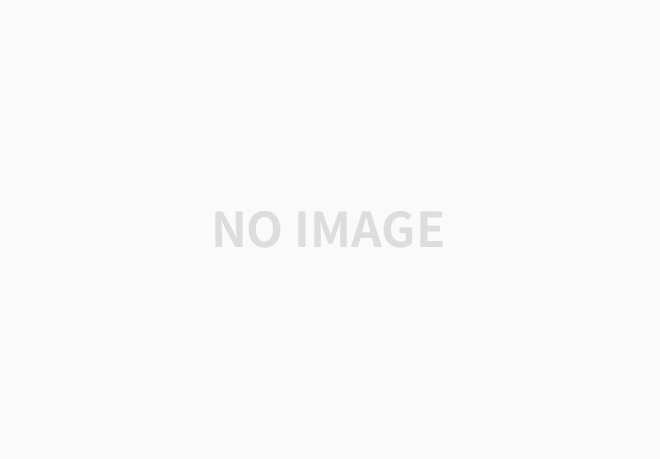
2. ThreadLocal 사용 예제
ThreadLocal은 쓰레드 단위로 실행되는 코드에서 동일한 객체를 참조하는 특징을 가지고 있습니다. 그렇기 떄문에 객체를 파라미티로 전달하지 않아도 같은 객체를 참조할 수 있게 됩니다.
ThreadLocal를 사용하는 대표적인 예는 다음과 같습니다.
- SpringSecurity에서 Authentication 정보
ThreadLocal의 SecurityContextHolder 영역에 인증에 필요한 Authentication 객체가 저장된다.
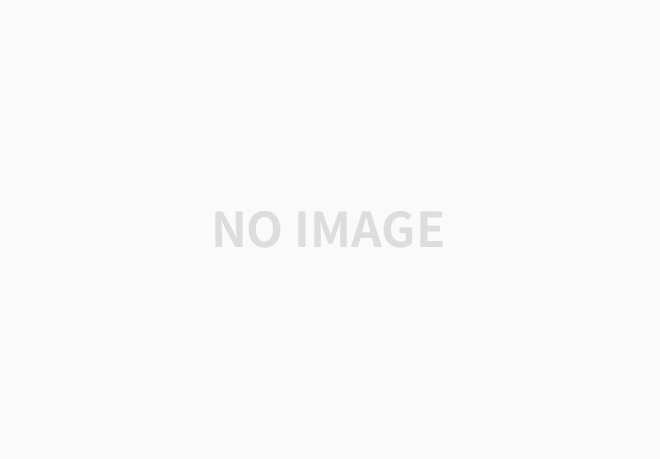
- TransactionalManager에서 Connection 관리
@Transactional 어노테이션을 붙이면 Spring AOP를 통해 트랜잭션 관리를 합니다.
아래 그림은 그것이 적용되는 과정인데요.
DB에 접근하기 위한 Connection 객체가 바로 ThreadLocal에 저장됩니다.
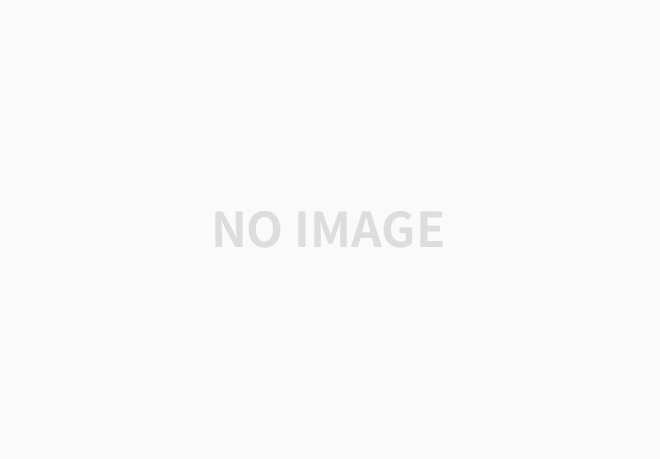
3. 주의사항
ThreadPool 환경에서 ThreadLocal를 사용할 경우 ThreadLocal의 데이터를 지우지 않고 반환하게 되면, 쓰레드가 재사용될 경우 잘못된 데이터가 들어갈 수 있습니다. 반드시 ThreadLocal 변수를 다 사용하고 나서 해당 데이터를 삭제해줘야합니다.
'JAVA' 카테고리의 다른 글
item 53) 가변인수는 신중히 사용하라 (0) | 2021.03.12 |
---|---|
HashMap 의 capacity와 load factor (0) | 2020.12.27 |
Java 멀티쓰레드 동기화 - (3) AtomicClass (0) | 2020.10.24 |
Java 멀티쓰레드 동기화 - (2) Synchronized (0) | 2020.10.11 |
Java 멀티쓰레드 동기화 - (1) Volatile (0) | 2020.10.03 |